Zustand
🐻 Bear necessities for state management in React
October 19, 2023
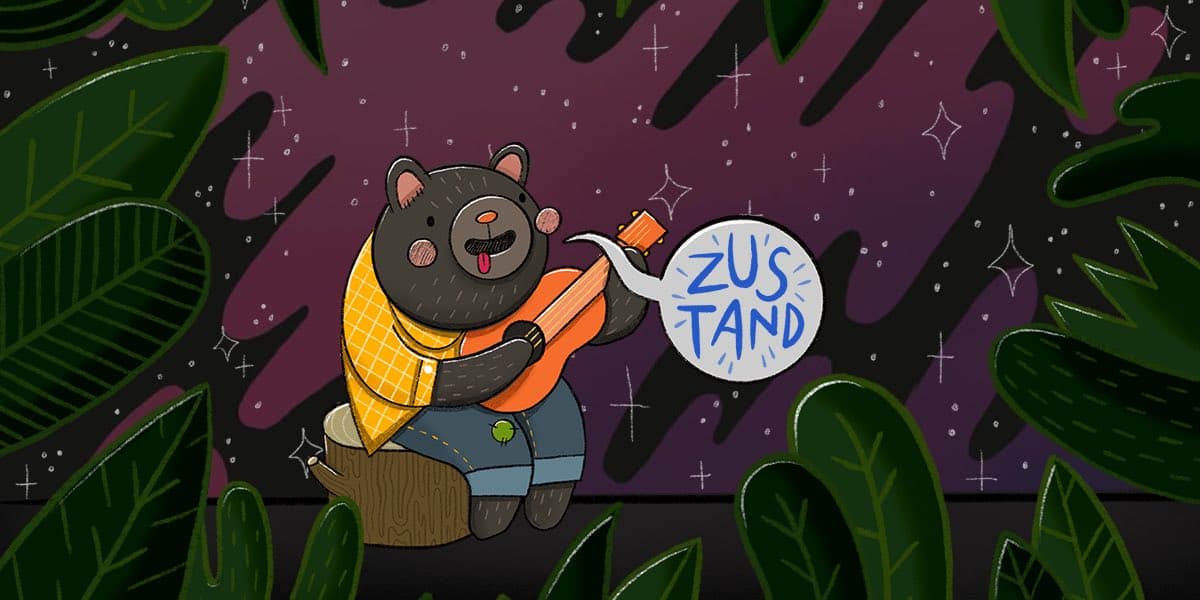
State management has always been an important part of modern applications. Over the years, various state management tools and libraries have emerged to help developers handle this complexity. First, Redux changed the game in larger applications with complex state logic. Especially back in the day, it was going hand in hand with React. But it has a heavy boilerplate and a steeper learning curve.
Then we had MobX, a state management solution based on reactive programming principles. It requires less boilerplate than Redux, automatically tracks state, and updates components. But it also had concepts such as observables can be difficult for some developers.
Then Facebook introduced the Context API which requires no additional libraries and integrates well with other React features. But it’s also not handy with complex state logic.
Examples can go on and on. So let’s cut to the chase.
In this article, I want to talk about a new state management library that has been gaining popularity recently - Zustand. I use it in my work and now we’ll take a closer look at Zustand and try to understand how it can simplify state management in React applications.
Let’s begin!
What is Zustand?
According to their official documentation:
A small, fast, and scalable bearbones state management solution. Zustand has a comfy API based on hooks. It isn't boilerplatey or opinionated, but has enough convention to be explicit and flux-like.
Zustand (which means "state" in German) is a minimalistic state management library for React and other JavaScript-based applications. It provides a way to create a global state without the added complexity of larger solutions, such as Redux or MobX. Zustand's API is straightforward and aims to be more React-ish, making it easier for developers familiar with React to pick up and use.
Here are some key features of Zustand:
- Simplicity: Zustand offers a simple API, which means a shorter learning curve, especially for those familiar with React's useState and useContext.
- No Redux Boilerplate: Unlike Redux, Zustand does away with the need for actions, types, reducers, and middleware for most use cases.
- Flexibility: It doesn't tie you to React, making it usable with other libraries or even vanilla JavaScript.
- Middlewares: Allows for middlewares to intercept actions and state changes.
- Devtools: Supports integration with React DevTools for a better debugging experience..
Getting Started with Zustand
To get started with Zustand, you’ll need to install it via npm or yarn:
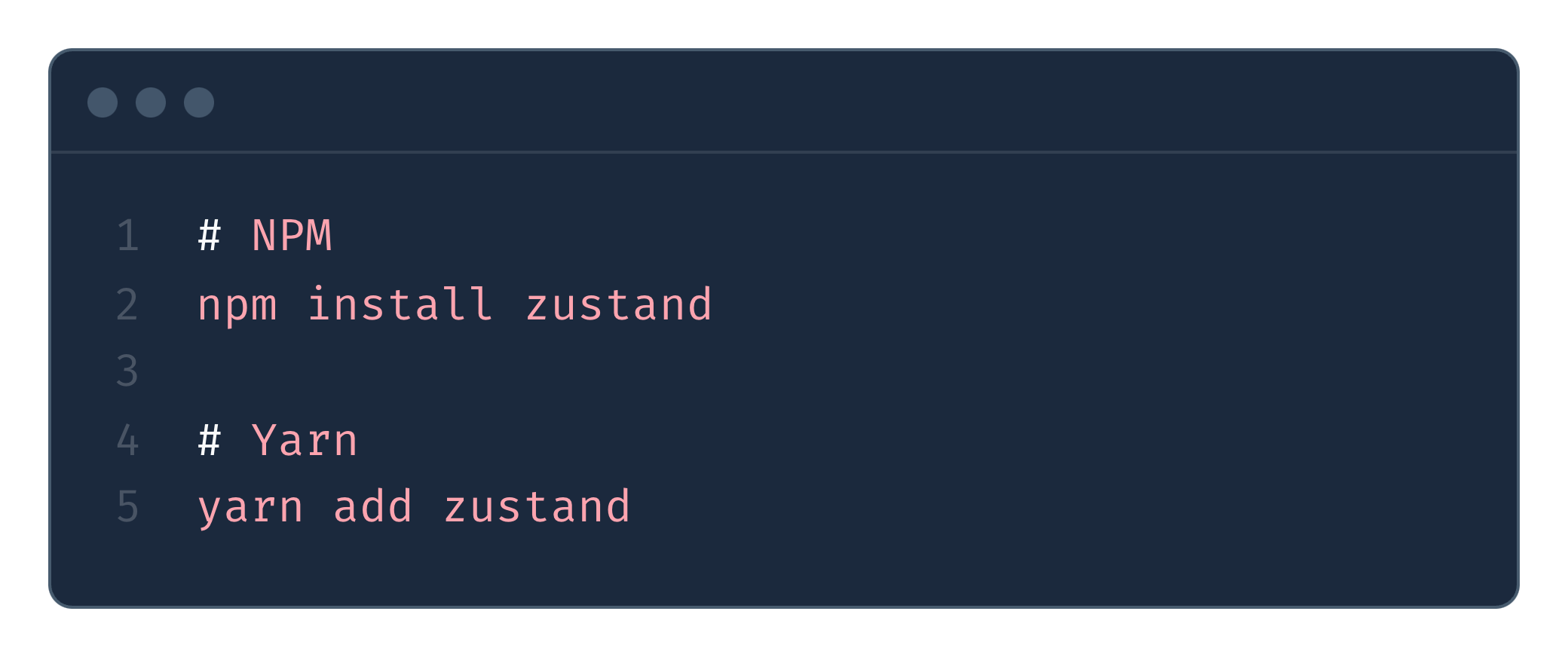
And here's a quick example to give you an idea of how it works:
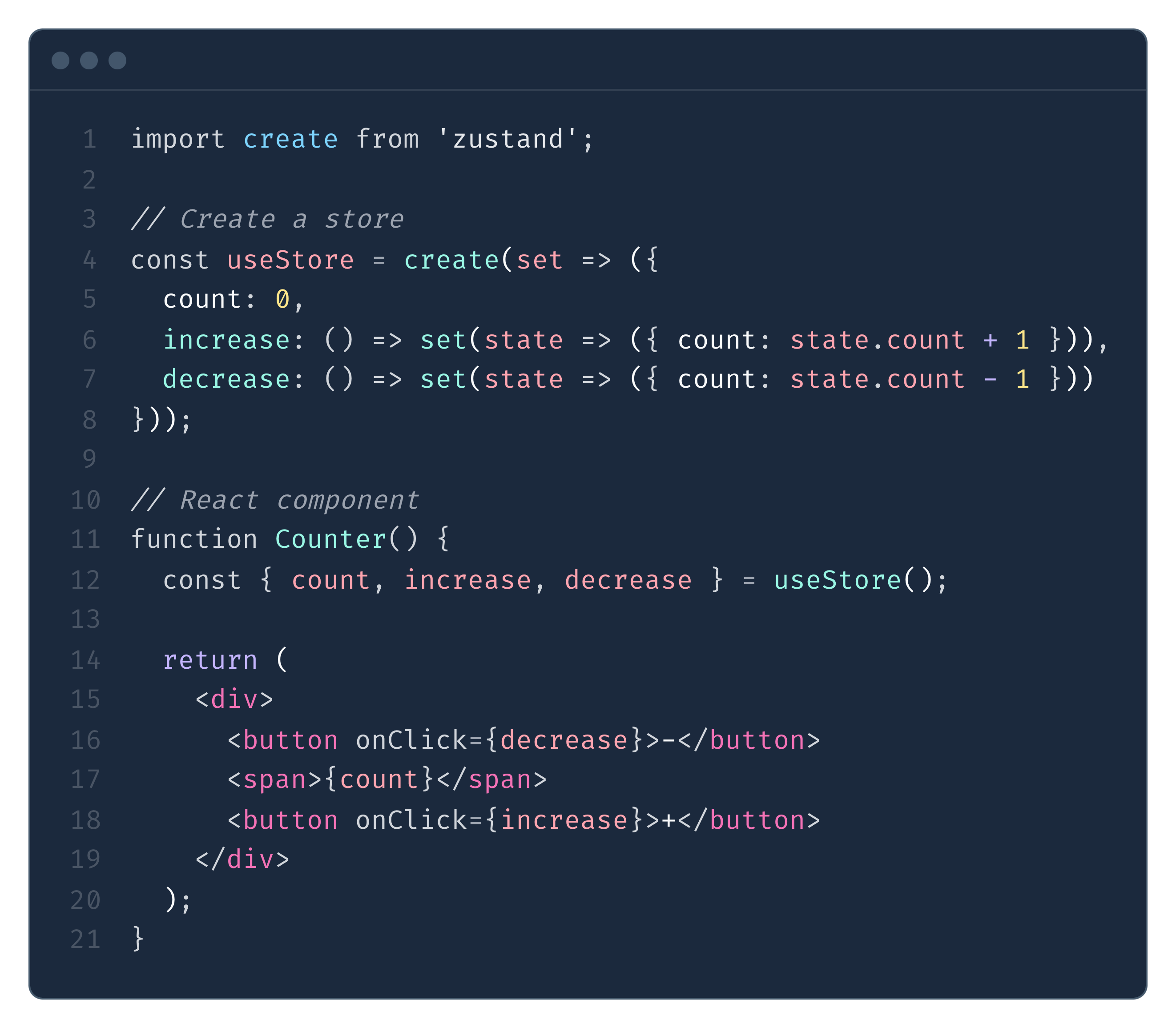
In the example above, create from Zustand is used to define a store with state and actions. This store is then utilized within a React component via the custom hook, useStore. It's simply a hook and you can use it anywhere, without the need for providers.
Pretty simple!
Here is the CodeSandbox example that you can play around.
Conclusion
Zustand provides a lightweight and powerful alternative to heavier state management solutions. It’s especially great for projects that need a simple global state.